t-richards
New member
Automated testing is a fundamental aspect of the development workflow for Rubyists. But how do you decide what to test? What happens when your tests run slowly and feel like they're just getting in the way? The following links are some resources which I have found to be helpful in answering these questions.
The mutant gem provides this functionality for Ruby, and is free for open source use.
github.com
The TestProf set of tools can help you identify bottlenecks in your test suite, as well as provide some guidance for how to fix the problems once you figure out where they are.
Minimal testing
In The Magic Tricks of Testing, Sandi Metz covers the secrets of writing stable tests and the philosophy of how to find the right testing balance.I am a testing minimalist. I want the lowest-cost testing option that can still provide me with some confidence.
Maximal testing
Mutation testing is the process of introducing intentional mistakes into your code to see whether your tests can catch them. As you continue down the path of mutation testing, you'll experience improvements for both your codebase and your test suite: you can eliminate code that is doing too much, as well as improve tests that aren't doing enough.I am a testing maximalist. Every line of code I write must have 100% test coverage, and then some. ...and not just the phony kind. I mean real honest coverage.
The mutant gem provides this functionality for Ruby, and is free for open source use.
GitHub - mbj/mutant: Automated code reviews via mutation testing - semantic code coverage.
Automated code reviews via mutation testing - semantic code coverage. - mbj/mutant
Faster tests
Slow tests are always frustrating, and there typically isn't a one-size-fits-all fix for this.My test suite is running slow. Like seriously many many hours. I have to parallelize it in CI otherwise it would take a full day to finish. What gives?
The TestProf set of tools can help you identify bottlenecks in your test suite, as well as provide some guidance for how to fix the problems once you figure out where they are.
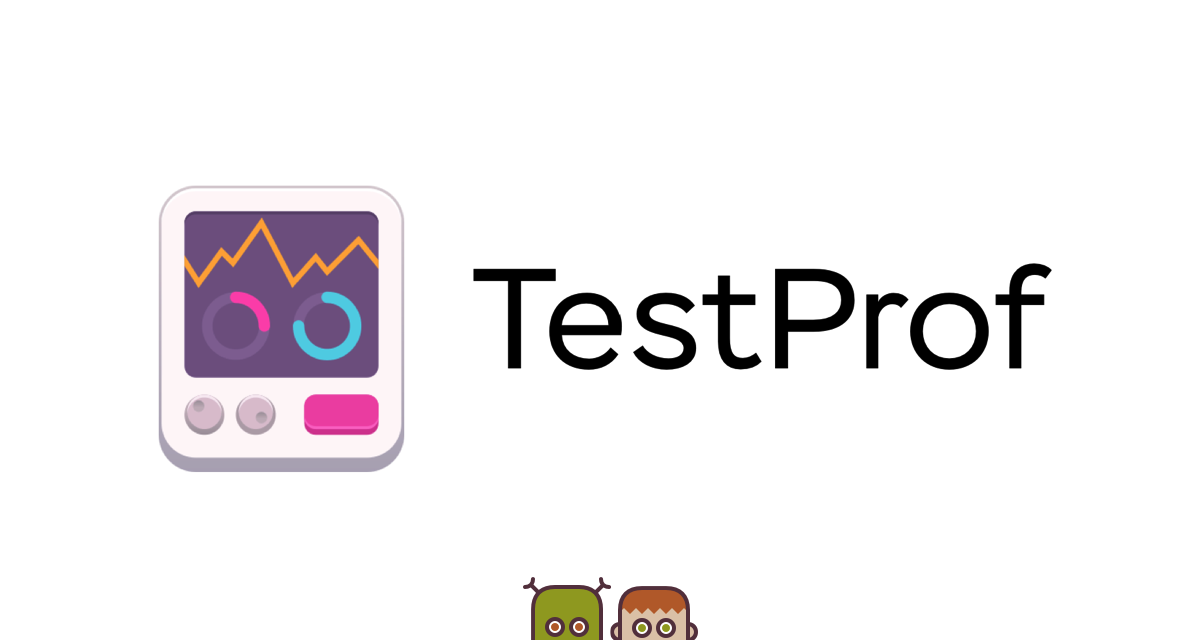